Table Of Content

We throw in a parameter that will give us the corresponding shape we wanted. Memento pattern is implemented with two Objects – originator and caretaker. The originator is the Object whose state needs to be saved and restored, and it uses an inner class to save the state of Object.
Java Design Patterns - Example Tutorial
How to use the flyweight design pattern in C# - InfoWorld
How to use the flyweight design pattern in C#.
Posted: Wed, 08 Jan 2020 08:00:00 GMT [source]
Use the Factory Method when you want to save system resources by reusing existing objects instead of rebuilding them each time. The first version of your app can only handle transportation by trucks, so the bulk of your code lives inside the Truck class. I hope, I have included enough information in this Java factory pattern example to make this post informative. So far we have design the classes need to be designed for making a CarFactory. Javatpoint provides tutorials with examples, code snippets, and practical insights, making it suitable for both beginners and experienced developers. Let’s say we have two sub-classes PC and Server with below implementation.
Final notes

Factory pattern introduces loose coupling between classes which is the most important principle one should consider and apply while designing the application architecture. Loose coupling can be introduced in application architecture by programming against abstract entities rather than concrete implementations. This not only makes our architecture more flexible but also less fragile. A Factory Pattern or Factory Method Pattern says that just define an interface or abstract class for creating an object but let the subclasses decide which class to instantiate.
Python Metaclass Tutorial with Examples - TechRepublic
Python Metaclass Tutorial with Examples.
Posted: Thu, 19 Oct 2023 07:00:00 GMT [source]
Factory Method Pattern
It is used when we have a super-class with multiple sub-classes and based on input, we want to return one of the sub-class. The Factory Design Pattern is a powerful technique for flexible object creation. By encapsulating the creation logic in a factory class, we achieve loose coupling and enhance the maintainability of our code.
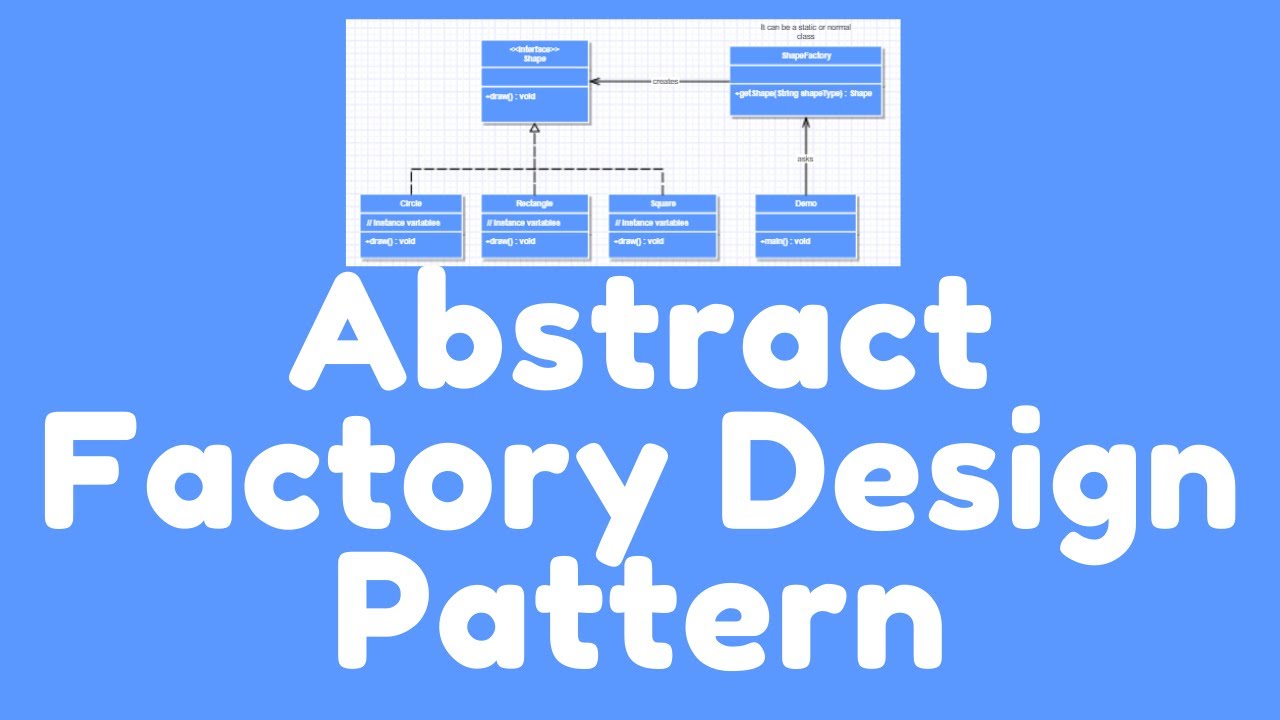
6 State Method
In Java, factory pattern is used to create instances of different classes of the same type. In my previous post, “Singleton design pattern in java“, we discussed various ways to create an instance of a class such that there can not exist another instance of same class in same JVM. I think the explanation in ‘Factory Design Pattern Advantages’ section is quite verbose.
In other words, subclasses are responsible to create the instance of the class. Structural design patterns are a subset of design patterns in software development that focus on the composition of classes or objects to form larger, more complex structures. They help in organizing and managing relationships between objects to achieve greater flexibility, reusability, and maintainability in a software system. To instantiate car objects, a GetFuelFactory class can be implemented. This class will have methods for creating different types of fuel based on the client’s request. Singleton Method is a creational design pattern, it provide a class has only one instance, and that instance provides a global point of access to it.
By delegating the responsibility of object creation to a factory, the pattern promotes loose coupling, enhances flexibility, and simplifies maintenance. The Factory Design Pattern is a design pattern that provides a single interface for creating objects, with the implementation of the object creation process being handled by a factory class. This factory class is responsible for instantiating objects based on a set of conditions or parameters that are passed to it by the client code. The Factory design pattern is intended to define an interface for creating an object, but allows subclasses to alter the type of objects that will be created. This pattern is particularly useful when the creation process involves complexity. The Factory Design Pattern is a creational design pattern that provides a simple and flexible way to create objects, decoupling the process of object creation from the client code.
That’s why we create a subclass for each dialog type and override their factory methods. The main idea is to define an interface or abstract class (a factory) for creating objects. Though, instead of instantiating the object, the instantiation is left to its subclasses. The Factory Method Pattern (also known as the Virtual Constructor or Factory Template Pattern) is a creational design pattern used in object-oriented languages. Each concrete product provides its own implementation of the getSymbol() method.
Strategy pattern is used when we have multiple algorithms for a specific task, and the client decides the actual implementation be used at runtime. We define multiple algorithms and let client applications pass the algorithm to be used as a parameter. The mediator design pattern is used to provide a centralized communication medium between different objects in a system. If the objects interact with each other directly, the system components are tightly-coupled with each other which makes maintainability cost higher and not flexible to extend easily. The mediator pattern focuses on providing a mediator between objects for communication and implementing loose-coupling between objects. The mediator works as a router between objects, and it can have its own logic to provide a way of communication.
The iterator pattern is one of the behavioral patterns and is used to provide a standard way to traverse through a group of objects. The iterator pattern is widely used in Java Collection Framework where the iterator interface provides methods for traversing through a Collection. This pattern is also used to provide different kinds of iterators based on our requirements. The iterator pattern hides the actual implementation of traversal through the Collection and client programs use iterator methods.
The composite pattern is used when we have to represent a part-whole hierarchy. When we need to create a structure in a way that the objects in the structure have to be treated the same way, we can apply the composite design pattern. A design pattern is a well-described solution to a common software problem. The factory typically has a single method called getTypeName() with the parameters you'd wish to pass.
Here is a simple test client program that uses above factory design pattern implementation. Super class in factory design pattern can be an interface, abstract class or a normal java class. For our factory design pattern example, we have abstract super class with overridden toString() method for testing purpose.
No comments:
Post a Comment